Last modified: Jan 10, 2023 By Alexander Williams
Understand How to Use django HttpResponseRedirect with Examples
Django HttpResponseRedirect is a subclass of HttpResponse that redirects the user to a specific URL.
In this tutorial, we'll cover Django HttpResponseRedirect, so pay attention and let's get started.
1. Using HttpResponseRedirect with examples
Before talking about why we need to use HttpResponseRedirect, let's write a simple view to understand how it's working.
So first, we need to import HttpResponseRedirect:
from django.http import HttpResponseRedirect
example #1:
views.py
#index def index(request): return HttpResponse("<h1>Index Page</h1>") #redirect user def redirect_user(request): return HttpResponseRedirect('/index')
urls.py
#index path('index/', index, name="index_page"), #redirect path('redirect/', redirect_user, name="redirect")
result:
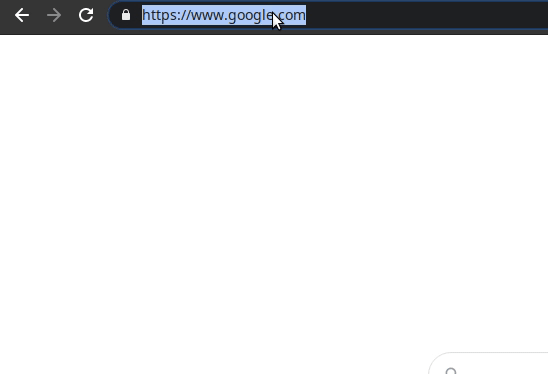
Let me explain:
We have 2 views index and redirect_user views, and the redirect_user view redirects us to index.
The question now is why we need HttpResponseRedirect?
For example, we want to redirect the user to a specific URL after submitting the form.
Example #2:
def redirect_user(request): if request.method == "POST": username = request.POST.get('username', None) password = request.POST.get('password', None) if username and password: return HttpResponseRedirect('/index') return render(request, "form.html")
result:
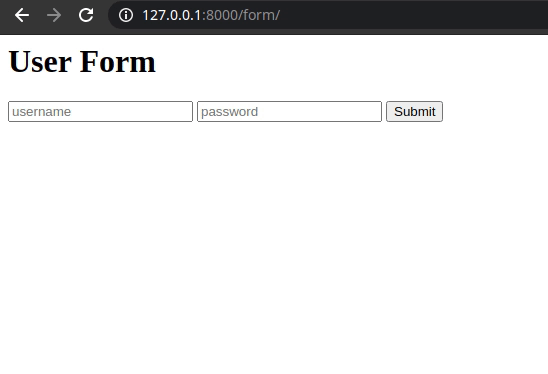
2. Django HttpResponseRedirect with Reverse function
If you want to use the URL tag instead of the URL, you should use the reverse function with HttpResponseRedirect.
Let's see an example.
urls.py
#index path('index/', index, name="index_page"),
views.py
def redirect_user(request): return HttpResponseRedirect(reverse('index_page'))
result:
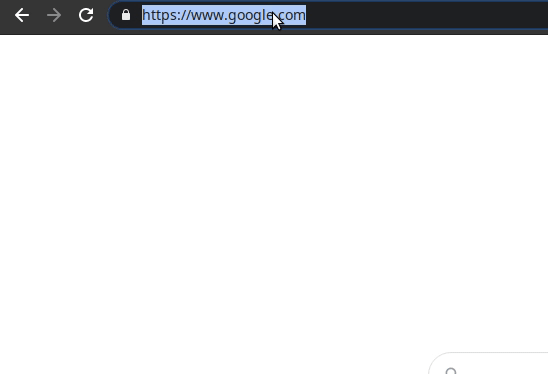
3. How to solve HttpResponseRedirect is not defined
issue output:
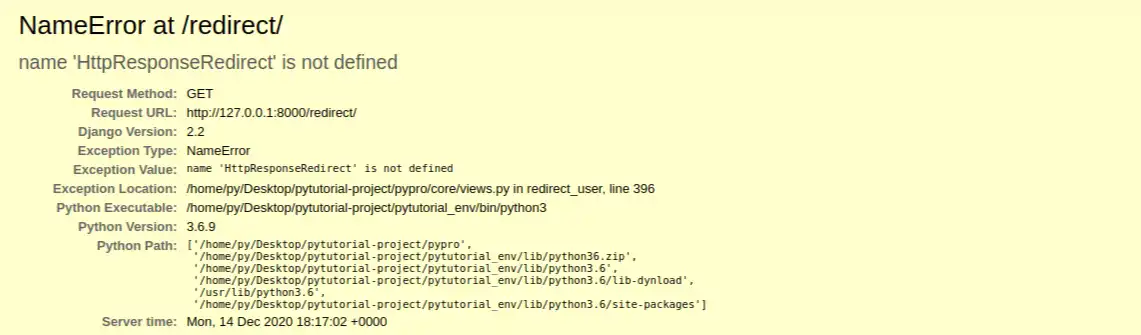
To solve this issue, you simply need to import the subclass like:
from django.http import HttpResponseRedirect