Last modified: Jan 10, 2023 By Alexander Williams
Django Admin: Add list_display, list_filter and search_fields
In this article, we'll learn how to Add list_display, list_filter, and search_fields in Django Admin Interface.
First, let's create a model that we'll work with it.
models.py
class EcoProduct(models.Model):
name = models.CharField(max_length=300)
price = models.DecimalField(max_digits=6, decimal_places=2)
category = models.CharField(max_length=300)
date = models.DateField(auto_now_add=True)
def __str__(self):
return self.name
Makemigration:
python manage.py makemigrations
migrate:
python manage.py migrate
Let's now register our model in admin.py.
#models
from .models import *
# Register your models here.
admin.site.register(EcoProduct)
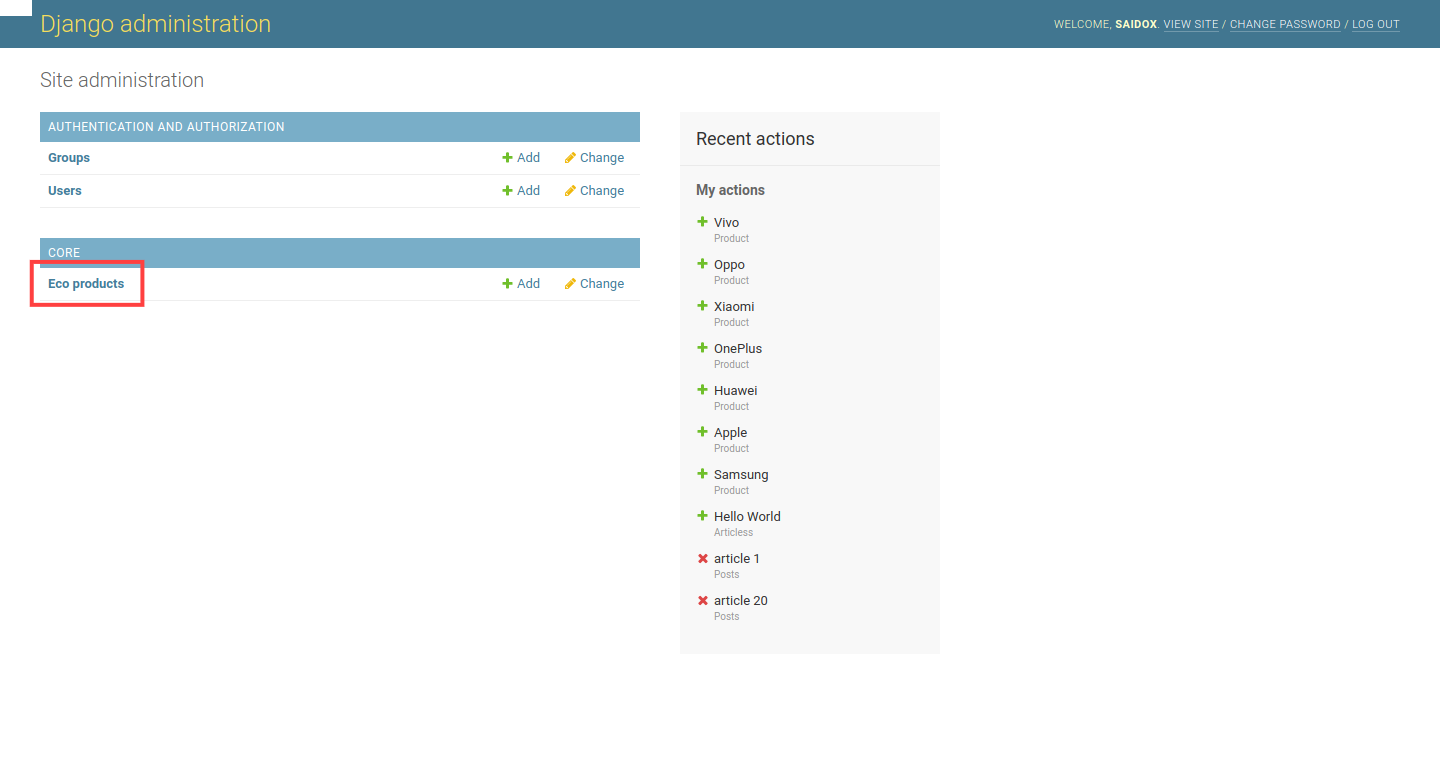
As you can see, our model has been registered, now let's add some products to our model.
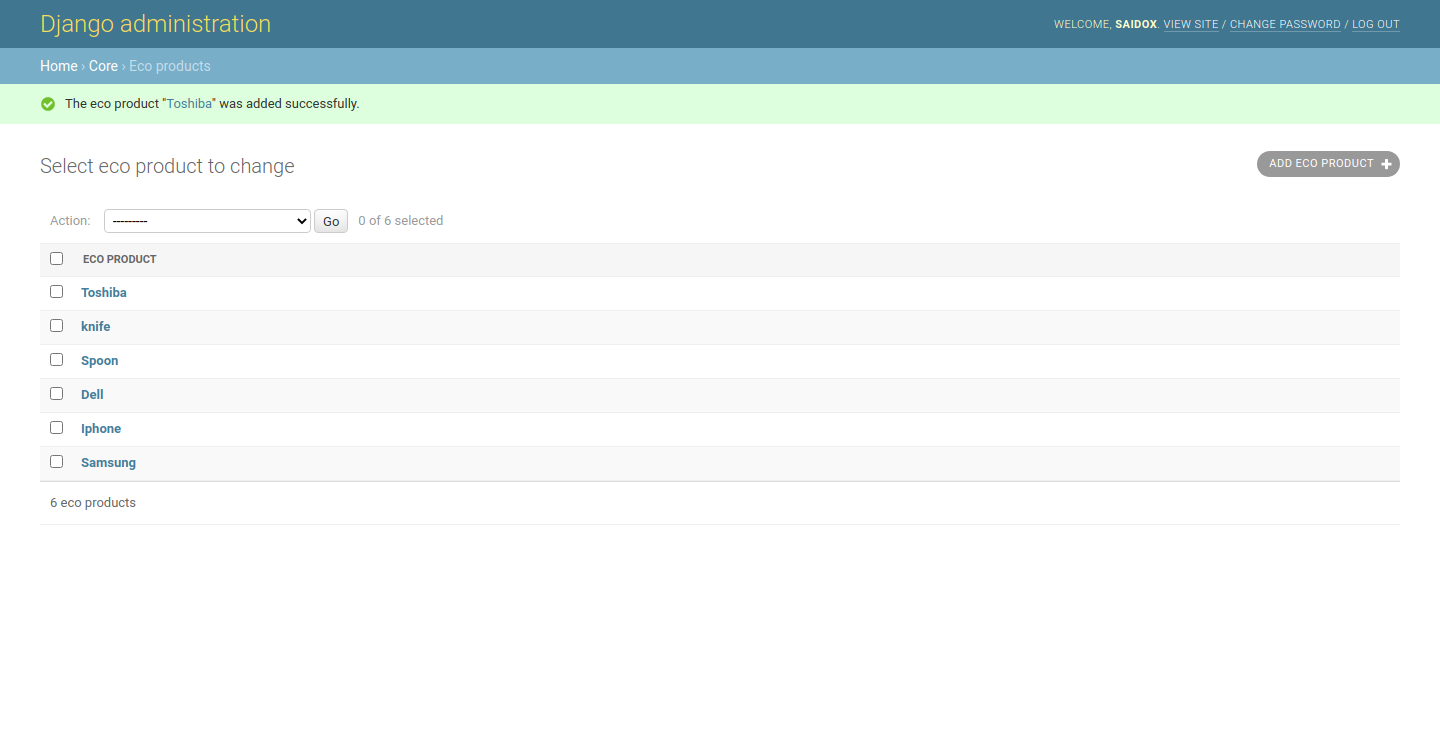
in this image, Django displayed just the field name that we have returned in the __str__ method.
Now, we'll add some options to our Django model admin like list_display, list_filter, and search_fields.
1. Adding list_display to Django Admin
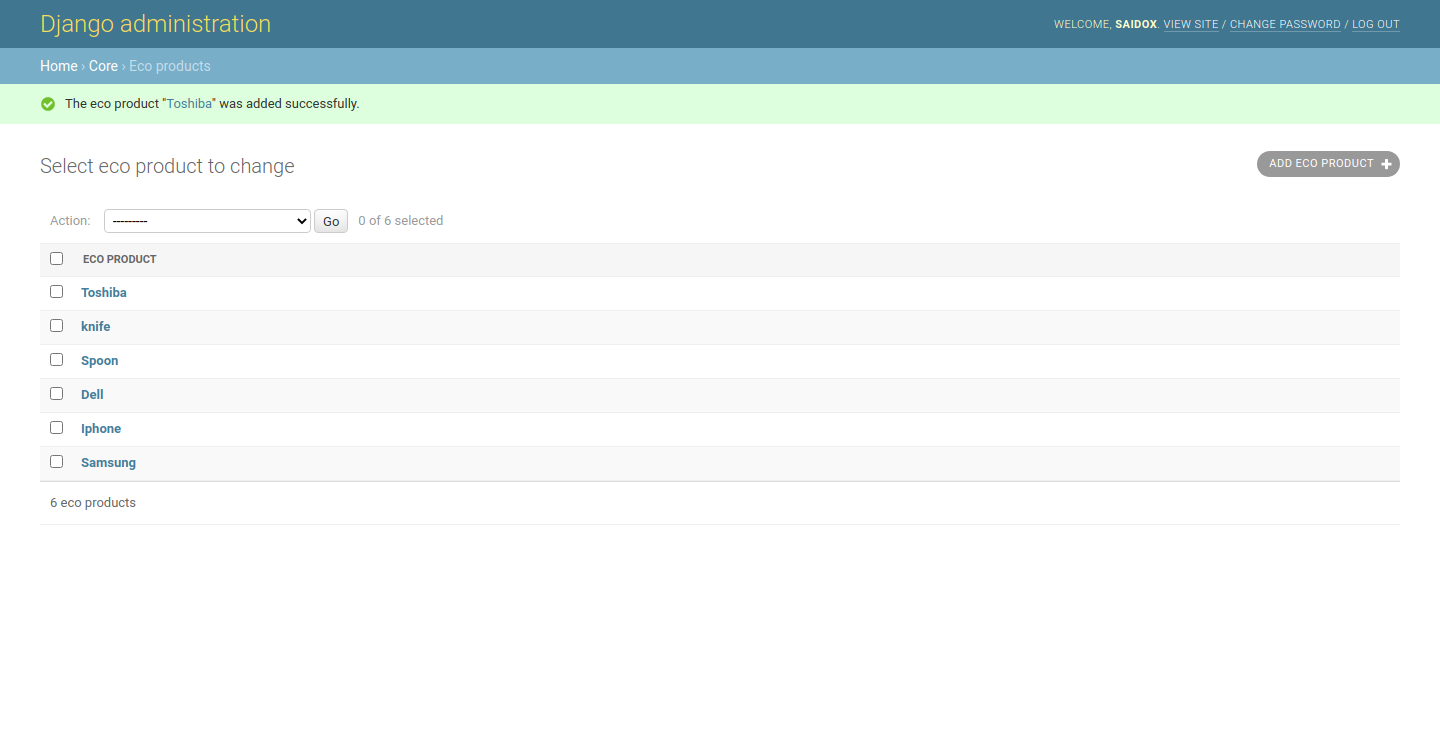
as yo can see in this image, just the name filed that is displayed, let's dispaly other fileds.
Let's dispaly other fileds.
syntax
list_display = ['Field']
admin.py
To display more fields, we need to change this code:
admin.site.register(EcoProduct)
to:
@admin.register(EcoProduct)
class EcoProductAdmin(admin.ModelAdmin):
list_display = ['name', 'price', 'category', 'date']
Result:
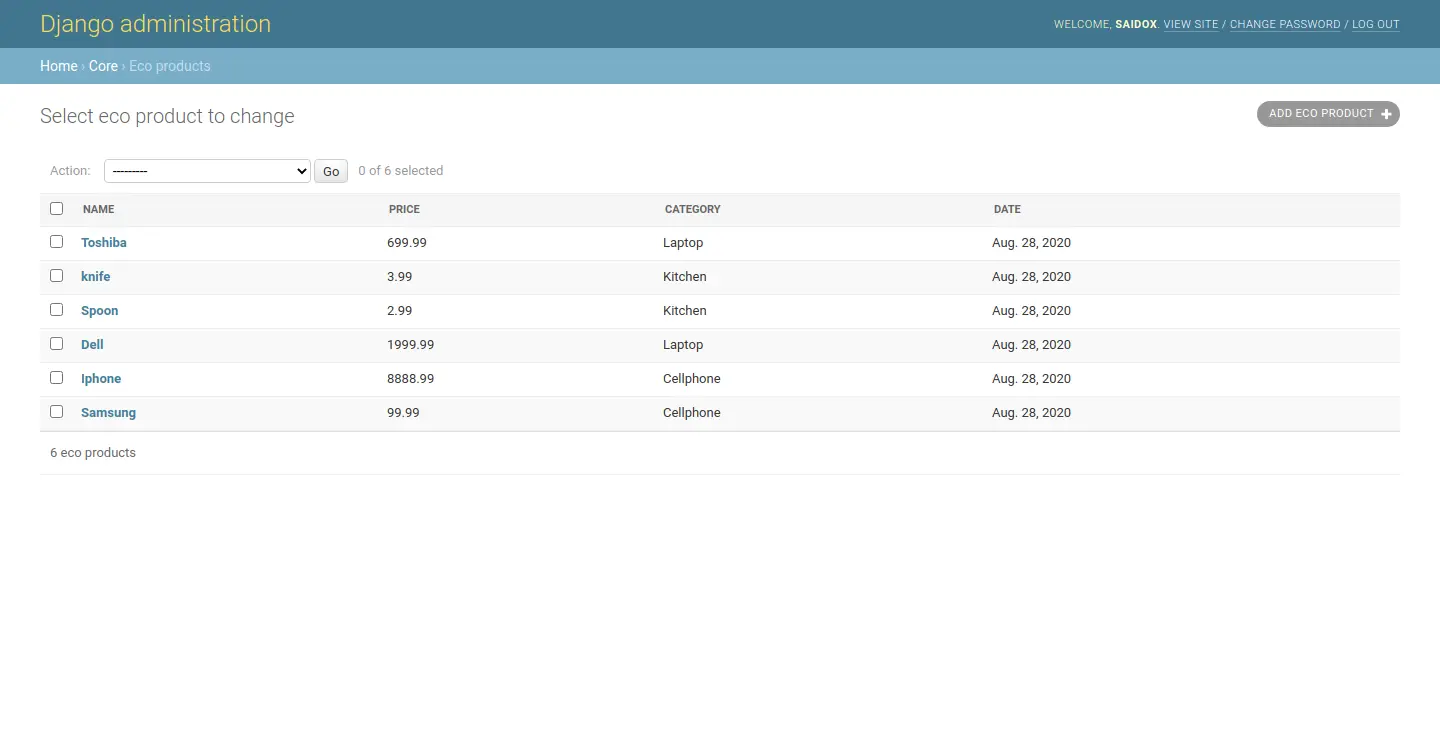
Done!
2. Adding list_filter to Django Admin
Let's say that we want to filter our model data by category & date, to do that, following the bellow code
Syntax
list_filter = ()
admin.py
@admin.register(EcoProduct)
class EcoProductAdmin(admin.ModelAdmin):
#list display
list_display = ['name', 'price', 'category', 'date']
#list Filter
list_filter = ('category','date')
result:
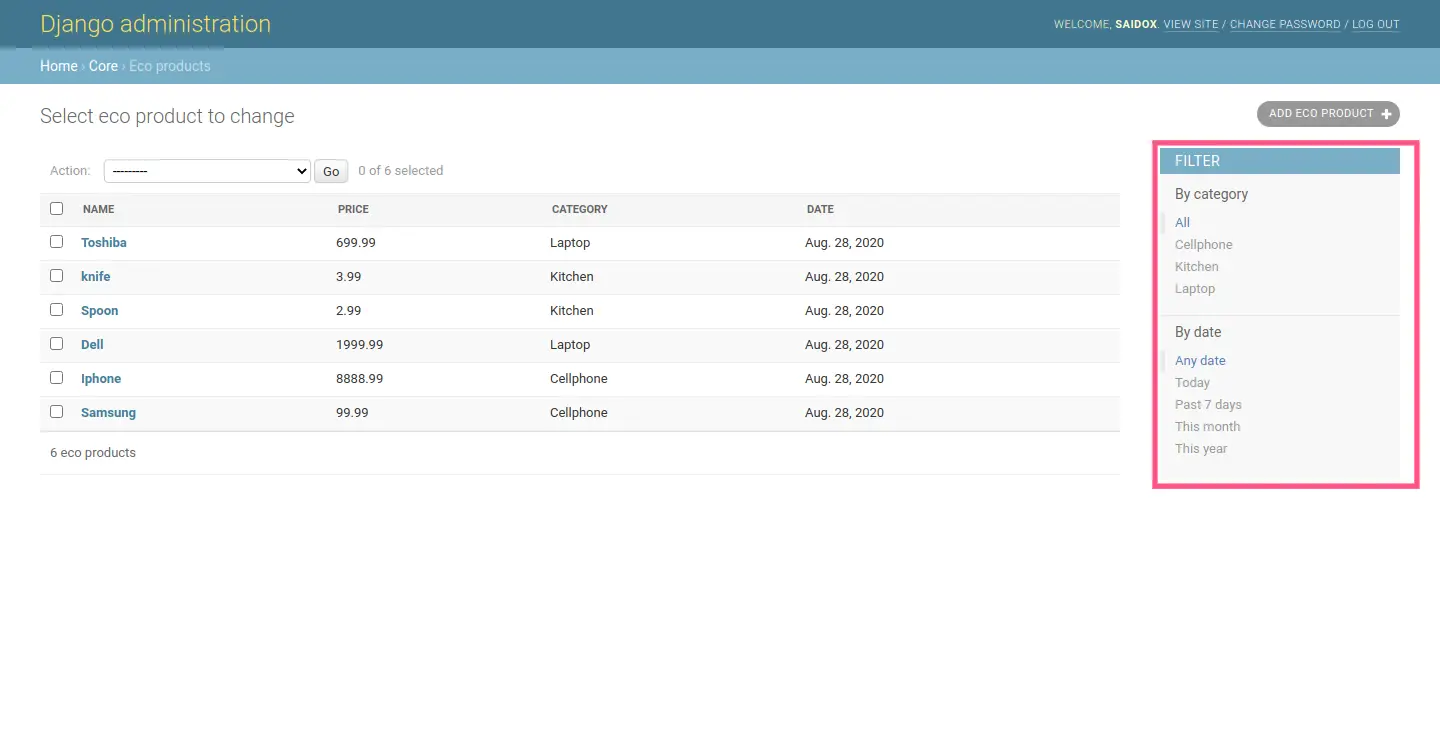
Let's try to filter by the Laptop category and see the result.
result:
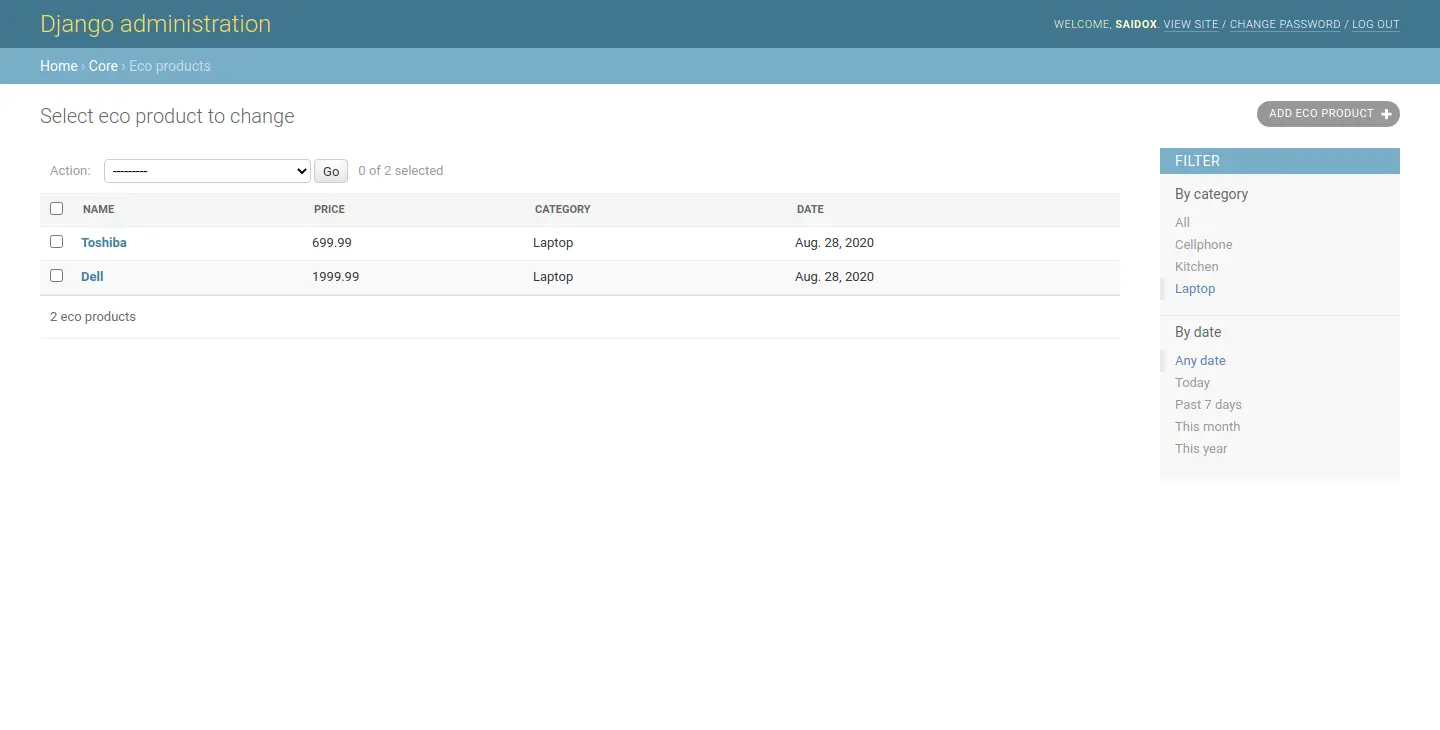
Great!
3. Adding search_fields to Django Admin Model
Now, we'll add search_fields to our Django admin that provides you search in your model data (by filed).
syntax
search_fields = ['field']
In our situation, we'll add the name field to search by name.
@admin.register(EcoProduct)
class EcoProductAdmin(admin.ModelAdmin):
#list display
list_display = ['name', 'price', 'category', 'date']
#list Filter
list_filter = ('category','date')
# search list
search_fields = ['name']
result:
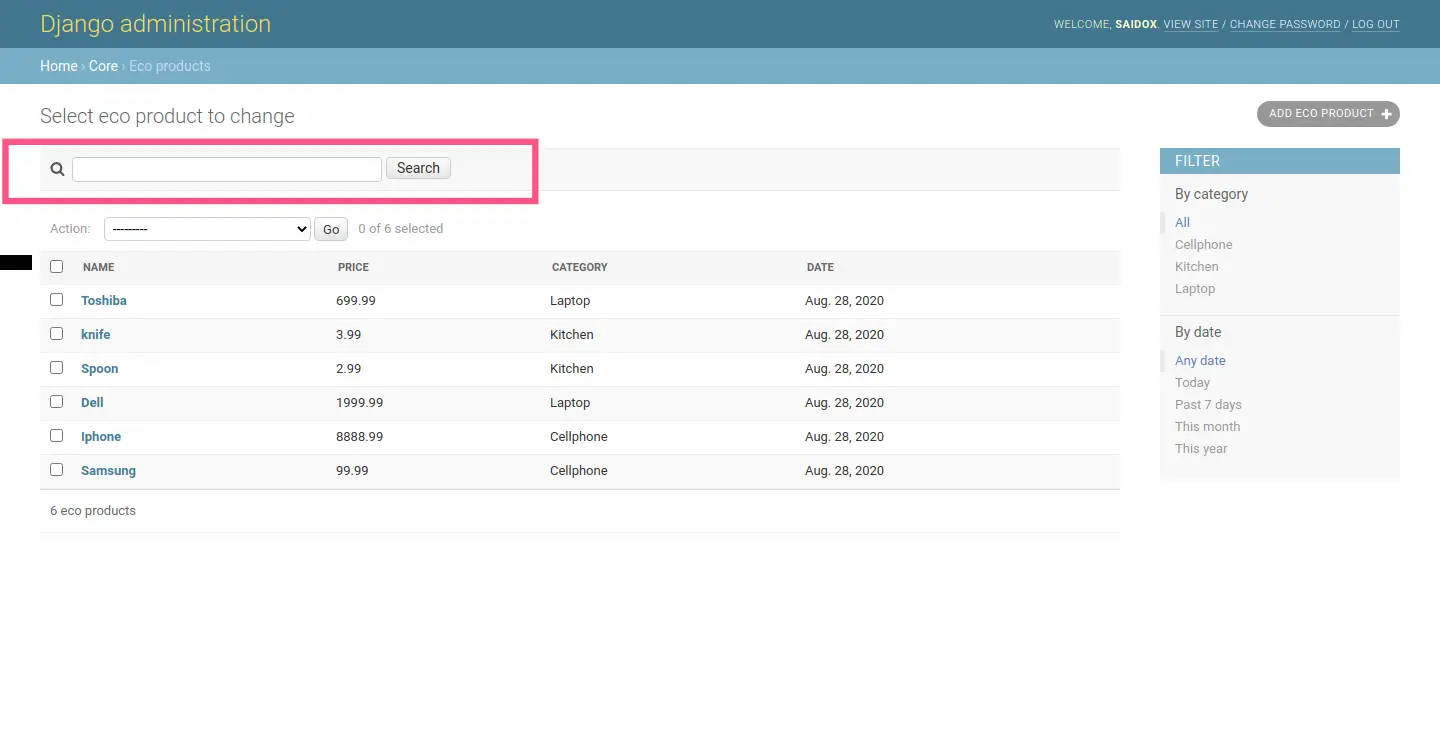
To test our Search field, let's try to search for Samsung.
result:
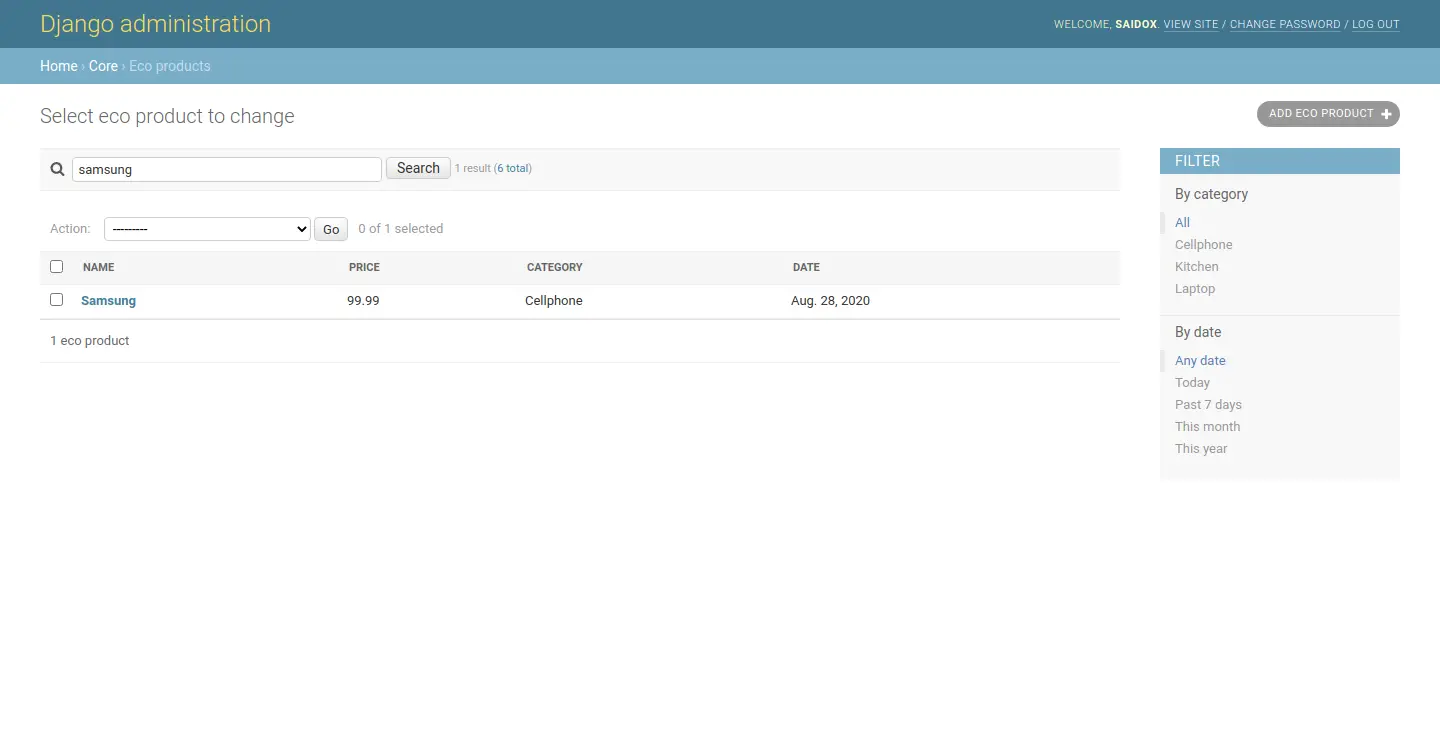
conclusion
So in this article, we have learned how to display multi fields in Django admin interface, we've also learned how to add filtering list and the search field to our Django admin for the models.
Finally, I'd like to recommend you to this article if you want to get a modern theme for your Django Admin interface How to Install and Set up Django Jet