Last modified: Jan 10, 2023 By Alexander Williams
How to Create a Simple Items Search Function in django
In this article, I'll show you how to create a search function to filter the database and return the result in the client side
Table Of Contents
Django Search Function database
First, we need to create a simple product model and add some data to it.
models.py
class Product(models.Model):
name = models.CharField(max_length=300)
price = models.DecimalField(max_digits=6, decimal_places=2)
def __str__(self):
return self.name
"makemigrations" and "migrate" our model:
python manage.py makemigrations
python manage.py migrate
now, let's add our model to the administration site.
admin.py
from django.contrib import admin
#models
from .models import *
# Register your models here.
admin.site.register(Product)
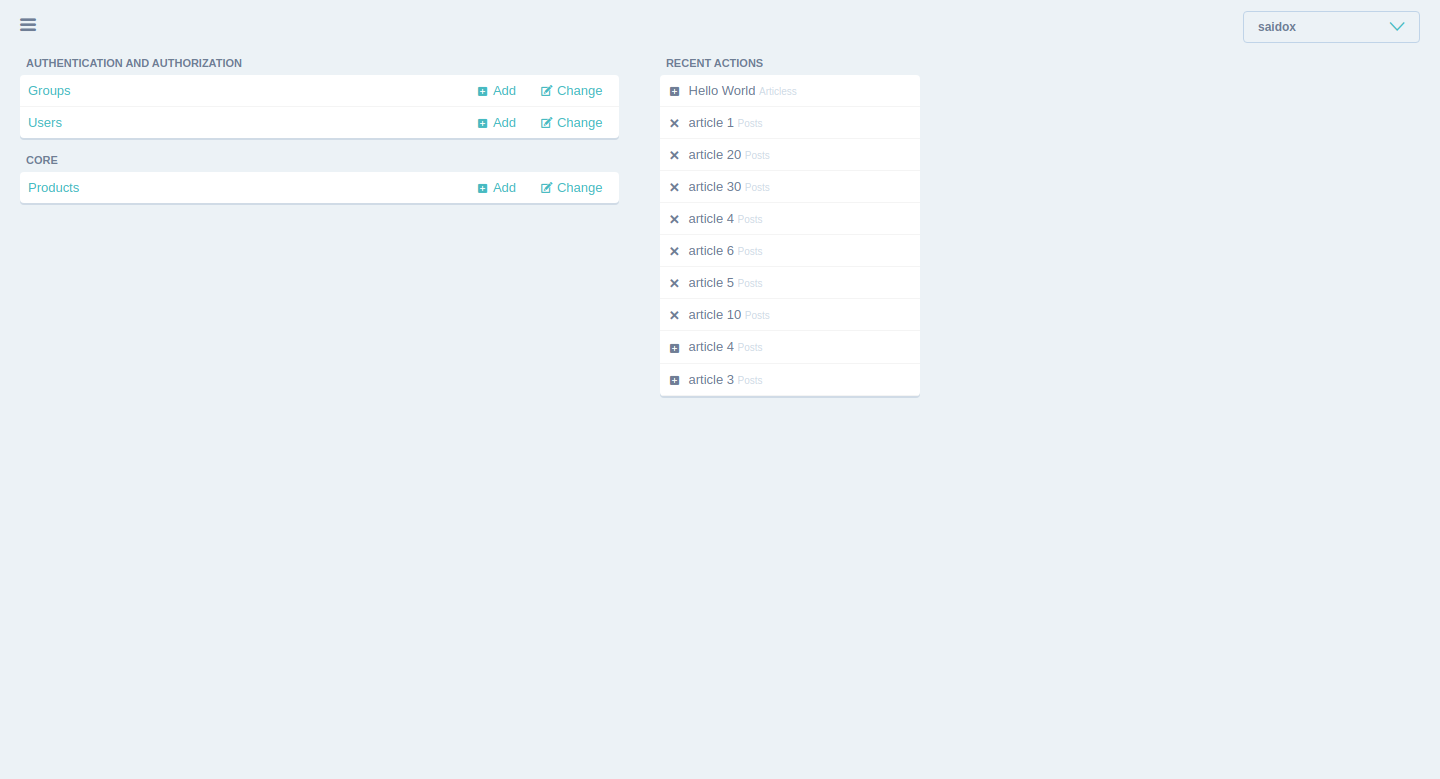
"if you want to have like my Django admin theme, visit this url How to Install and Set up Django Jet "
let's add some data to our model
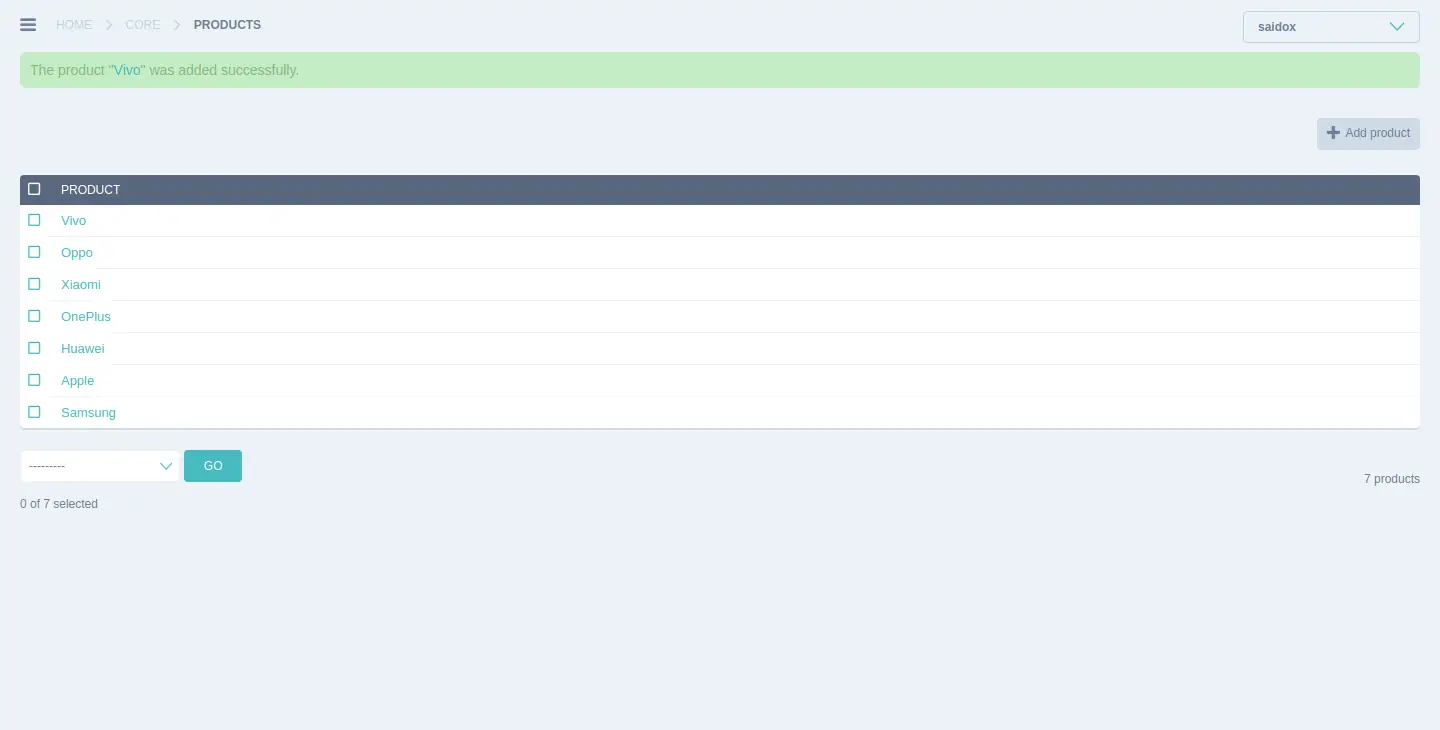
Creating our Django search function
first, we'll import our product model then create the search function.
views.py
#models.py
from .models import *
#views
def search_product(request):
""" search function """
if request.method == "POST":
query_name = request.POST.get('name', None)
if query_name:
results = Product.objects.filter(name__contains=query_name)
return render(request, 'product-search.html', {"results":results})
return render(request, 'product-search.html')
the important line is:
result = Product.object.filter(name__contains=query_name)
Its means retrieve all product that contains query_name.
Preparing our Django search client-side
Now, we need to create "product-search.html" in our templates folder.
product-search.html
<!DOCTYPE html>
<html>
<head>
<title>Django Search</title>
</head>
<body>
<form action="{% url 'search' %}" method="POST">
{% csrf_token %}
<input type="text" name="name">
<input type="submit" name="submit" value="Search">
</form>
{% for result in results %}
<p>{{result.name}}</p>
{% endfor %}
</body>
</html>
{% url 'search' %} the name that we have set as the name of the path in url.py
result:
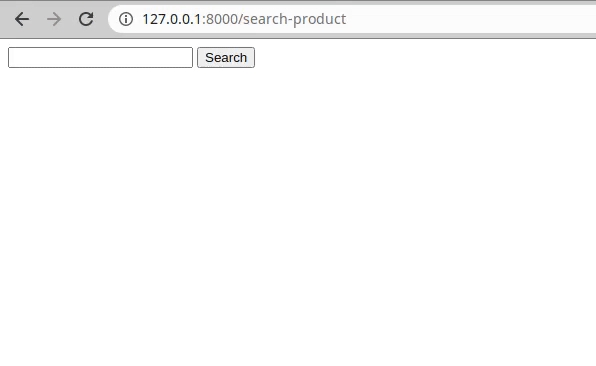
Happy Coding!