Last modified: Jan 10, 2023 By Alexander Williams
Python String Uppercase | Check if Uppercase Exists
In this tutorial, we'll learn how to check if a python string contains uppercase by using the isupper() method and regex.
Table Of Contents
1. Checking if Python string contains uppercase using isupper() method
The isupper() method return True if all of the string's letter is uppercase, otherwise, it returns False.
So we'll use the method to check each letter of string if it is uppercase.
Example 1: Not recommended
this example is not recommended, it's just to understand how it works.
#Not recommended
#string
string = "hello Python"
#loop over the string
for l in string:
#check if the string's letter is uppercase
if l.isupper() is True:
print("Yes, the string has uppercase")
break
So first, we iterated over the string, then checked letters one by one, If a letter is uppercase, the code will print the message and will break the loop.
output:
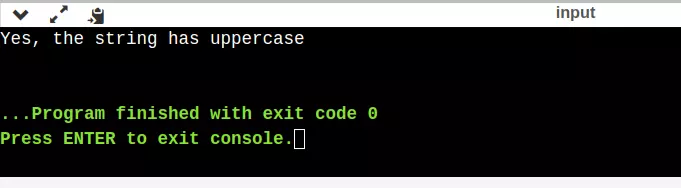
as you can see, the string has uppercase P.
Example 2: Recommended
In the second example, we'll do the same way that we've done in the first example by using any() method.
The any() function returns True if any item in a lopping is True and if not returns False.
example:
#Recommended
#string
string = "hello Python"
print(any(l.isupper() for l in string))
output:
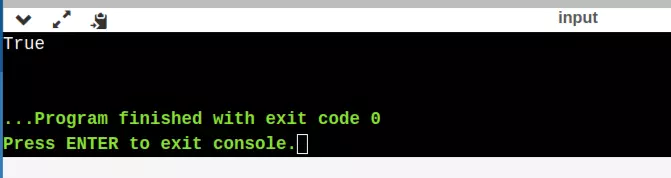
As you can see, it returned True.
2. Checking if Python string contains uppercase using Regex method
Regex is another way to check if a string contains uppercase.
example:
# find uppercase
find_upper = re.findall('[A-Z]', string)
#check if list has items
if find_upper:
print("Yes, the string has uppercase")
First, we tried to find an uppercase letter by using regex syntax, then checked if the list had items, if so, that means the string had an uppercase letter.
output:
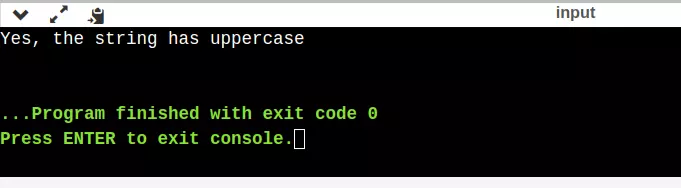