Last modified: Jan 10, 2023 By Alexander Williams
How to Minify or Compress HTML in Python
In this tutorial, I'm going to show you how to minify HTML using the htmlmin library.
Let's get started.
Install the htmlmin library
Install htmlmin via pip:
pip install htmlmin
Quick start
The code below will minify a simple HTML string.
import htmlmin
# HTML Text
html = '''
<p>In this tutorial, we'll learn how to turn or Convert a List into a Tuple in python.</p>
<!-- Comment-->
<div class="table-of-content p-4 p-md-5">
<h4>Contents</h4>
<ul>
<li class="toc2">
<a href="#1"><span class="number">1.</span> Method 1: turn a List to a Tuple using tuple() built-in function</a>
</li>
<li class="toc2">
<a href="#2"><span class="number">2.</span> Method 2: turn a List to a Tuple using for loop</a>
</li>
</ul>
</div>
'''
#minify
minified = htmlmin.minify(html)
print(minified)
output
<p>In this tutorial, we'll learn how to turn or Convert a List into a Tuple in python.</p><div class="table-of-content p-4 p-md-5"><h4>Contents</h4><ul><li class=toc2><a href=#1><span class=number>1.</span> Method 1: turn a List to a Tuple using tuple() built-in function</a></li><li class=toc2><a href=#2><span class=number>2.</span> Method 2: turn a List to a Tuple using for loop</a></li></ul></div>
Minify multiple HTML text
To minify multiple HTML text, we'll use the Minifier class.
import htmlmin
html = '''
<p>In this tutorial, we'll learn how to turn or Convert a List into a Tuple in python.</p>
<!-- Comment-->
<div class="table-of-content p-4 p-md-5">
<h4>Contents</h4>
<ul>
<li class="toc2">
<a href="#1"><span class="number">1.</span> Method 1: turn a List to a Tuple using tuple() built-in function</a>
</li>
<li class="toc2">
<a href="#2"><span class="number">2.</span> Method 2: turn a List to a Tuple using for loop</a>
</li>
</ul>
</div>
'''
html2 = '''
<p>In this tutorial, we'll learn how to turn or Convert a List into a Tuple in python.</p>
<!-- Comment-->
<div class="table-of-content p-4 p-md-5">
<h4>Contents</h4>
<ul>
<li class="toc2">
<a href="#1"><span class="number">1.</span> Method 1: turn a List to a Tuple using tuple() built-in function</a>
</li>
<li class="toc2">
<a href="#2"><span class="number">2.</span> Method 2: turn a List to a Tuple using for loop</a>
</li>
</ul>
</div>
'''
#minify multiple
minified = htmlmin.Minifier().minify(html, html2)
print(minified)
output
<p>In this tutorial, we'll learn how to turn or Convert a List into a Tuple in python.</p> <!-- Comment--> <div class="table-of-content p-4 p-md-5"> <h4>Contents</h4> <ul> <li class=toc2> <a href=#1><span class=number>1.</span> Method 1: turn a List to a Tuple using tuple() built-in function</a> </li> <li class=toc2> <a href=#2><span class=number>2.</span> Method 2: turn a List to a Tuple using for loop</a> </li> </ul> </div> <p>In this tutorial, we'll learn how to turn or Convert a List into a Tuple in python.</p> <!-- Comment--> <div class="table-of-content p-4 p-md-5"> <h4>Contents</h4> <ul> <li class=toc2> <a href=#1><span class=number>1.</span> Method 1: turn a List to a Tuple using tuple() built-in function</a> </li> <li class=toc2> <a href=#2><span class=number>2.</span> Method 2: turn a List to a Tuple using for loop</a> </li> </ul> </div>
Minify HTML file and save it
Now, we'll minify an HTML file and save the output on the same file.
import htmlmin
#minify
with open('/home/py/Desktop/py/tutorials/html.html', 'r') as f:
minified = htmlmin.minify(f.read())
#write
with open('/home/py/Desktop/py/tutorials/html.html', 'w') as f:
f.write(minified)
html.html before:
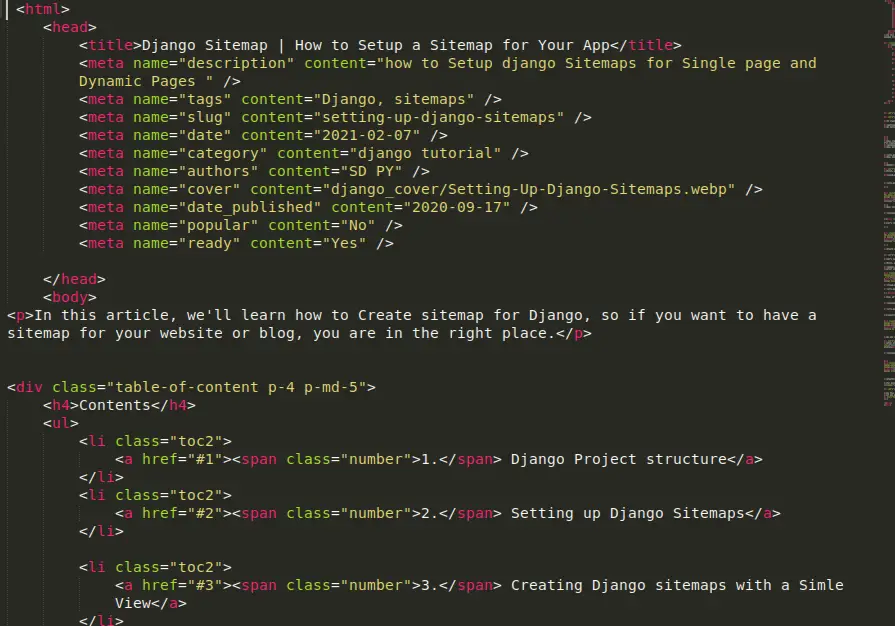
html.html after:
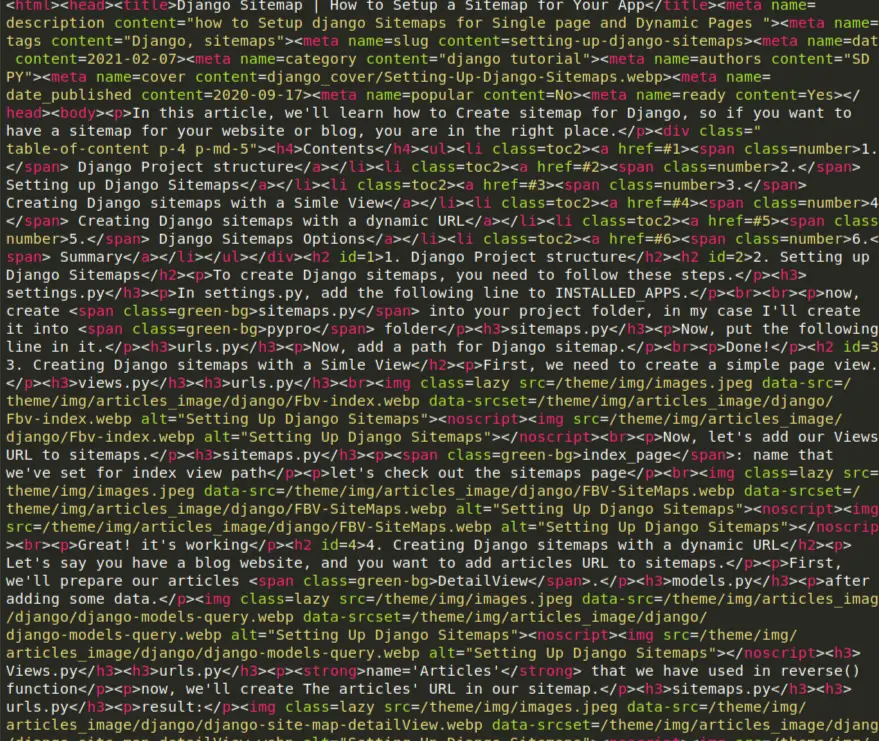
htmlmin parameters
- remove_comments=True/False,
- remove_empty_space=True/False,
- remove_all_empty_space=True/False,
- reduce_empty_attributes=True/False,
- reduce_boolean_attributes=True/False,
- remove_optional_attribute_quotes=True/False,
- convert_charrefs=True/False,
- keep_pre=True/False,