Last modified: Jan 10, 2023 By Alexander Williams
How to make a pagination with django and ajax/jquery
In this article, we'll code a simple Django pagination by using ajax/jquery.
Update: Django rest Framework has been removed in this example
example:
models.py
class Articles(models.Model):
title = models.CharField(max_length=300)
def __str__(self):
return self.title
views.py
from django.shortcuts import render
from django.http import JsonResponse
from django.core.paginator import Paginator
#models.py
from .models import Articles
def pagination_pro(request):
#model
my_model = Articles.objects.all()
#number of items on each page
number_of_item = 10
#Paginator
paginatorr = Paginator(my_model, number_of_item)
#query_set for first page
first_page = paginatorr.page(1).object_list
#range of page ex range(1, 3)
page_range = paginatorr.page_range
context = {
'paginatorr':paginatorr,
'first_page':first_page,
'page_range':page_range
}
#
if request.method == 'POST':
page_n = request.POST.get('page_n', None) #getting page number
results = list(paginatorr.page(page_n).object_list.values('id', 'title'))
return JsonResponse({"results":results})
return render(request, 'index.html',context)
urls.py
from django.urls import path
from core.views import *
urlpatterns = [
path('pagination/', pagination_pro, name='pagination_p'),
]
index.html
<html> <head> <title>How to make a pagination with django and jquery</title> <script src="https://code.jquery.com/jquery-3.4.1.min.js" integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo=" crossorigin="anonymous"></script> </head> <body> <!-- queryset for first page --> <div id="posts"> {% for i in first_page %} <h2>{{i.title}}</h2> {% endfor %} </div> <!-- loop pagination --> {% for i in page_range %} <a style="margin-left: 5px; font-size: 20px;" href="{{i}}">{{i}}</a> {% endfor %} <script> $('a').click(function(event){ event.preventDefault(); var page_n = $(this).attr('href'); // ajax $.ajax({ type: "POST", url: "{% url 'pagination_p' %}", // name of url data : { page_n : page_n, //page_number csrfmiddlewaretoken: '{{ csrf_token }}', }, success: function (resp) { //loop $('#posts').html('') $.each(resp.results, function(i, val) { //apending posts $('#posts').append('<h2>' + val.title + '</h2>') }); }, error: function () {} }); // }); </script> </body></html>
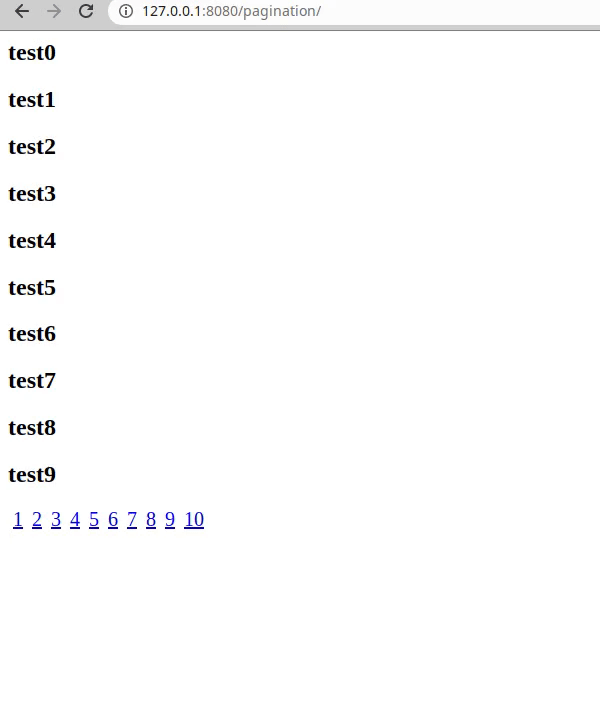