Last modified: Jan 10, 2023 By Alexander Williams
How to Submit a Django Form With Vuejs and Axios
In this article, I'd like to share with you how to submit a Django form with Vuejs.
So in this example, we'll write a simple form with custom validations.
Table Of Contents
1. In views.py
from django.shortcuts import render
from django.http import JsonResponse
import json
def submit_form(request):
if request.method == "POST":
data = json.loads(request.body)
username = data['username']
password = data['password']
if username and password:
response = f"Welcome {username}"
return JsonResponse({"msg":response}, status=201)
else:
response = "username or password is empty"
return JsonResponse({"err":response}, status=400)
return render(request, 'submit-form.html')
I think the above code doesn't need to explain
2. In urls.py
In urls.py we'll add path for our view function. Name="Submitform" is the name of the path that we'll use in our Axios Url.
#submit form django vuejs
path('submit-form', submit_form, name="submitform")
3. In submit-form.html
In submit-form.html, first, we need to add CDN of Vuejs and Axios, then create our Vuejs app and submit method.
<!DOCTYPE html> <html> <head> <title>Django Vuejs</title> </head> <body> <div id="app"> <p style="color: green">[[success_msg]]</p> <p style="color: red">[[err_msg]]</p> <!-- form --> <form> <input type="text" v-model="username"> <input type="password" v-model="password"> <input v-on:click.prevent="submitForm" type="submit" value="submit"> </form> </div> <!-- vuejs and axios --> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script src="https://unpkg.com/axios/dist/axios.min.js"></script> <script> const vms = new Vue({ delimiters: ['[[', ']]'], el: '#app', data: { username: null, password: null, success_msg: "", err_msg: "", }, methods: { submitForm: function(){ this.success_msg = "" this.err_msg = "" axios({ method : "POST", url:"{% url 'submitform' %}", //django path name headers: {'X-CSRFTOKEN': '{{ csrf_token }}', 'Content-Type': 'application/json'}, data : {"username":this.username, "password":this.password},//data }).then(response => { this.success_msg = response.data['msg']; }).catch(err => { this.err_msg = err.response.data['err']; }); }, }, }); </script> </body> </html>
4. Result
This is how our result looks like after submitting the form:
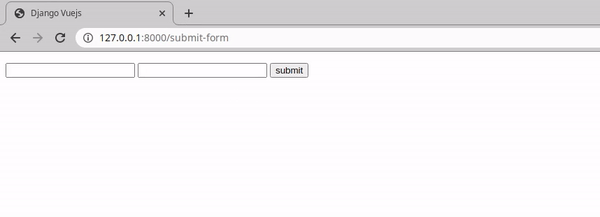