Last modified: Jan 10, 2023 By Alexander Williams
Understanding Django UpdateView With Simple Example
In this tutorial, we'll learn how to use Django UpdateView with a simple example.
So, UpdateView is the CBV that will create and edit forms.
In this example, we'll update a model's records dynamically.
1. Creating a page list's records
Before using UpdateView, we need to display a model's Records that we'll update it.
models.py
So, let's create a simple Posts model.
class Posts(models.Model):
title = models.CharField(max_length=300, unique=True)
time = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
views.py
To display the Posts' records, we need to add the following lines to our Views.py.
from django.views.generic.list import ListView
class Posts_list(ListView):
model = Posts #model
fields = '__all__' # fields
template_name = 'posts-list.html'
urls.py
Now, we need to add a path for our posts' list view.
#posts-list
path('posts-list', Posts_list.as_view(), name="posts-list"),
2. Updating records using Django UpdateView
In this part, we'll write a dynamic page to update our Posts record.
views.py
In our views, we need to add the following lines.
#UpdateView
from django.views.generic.edit import UpdateView
class Update(UpdateView):
model = Posts #model
fields = ['title'] # fields / if you want to select all fields, use "__all__"
template_name = 'update_form.html' # templete for updating
success_url="/posts-list" # posts list url
model: The model that you want to modify.
fields: The model's fields that you want to display in the form.
template_name: The template's name in that you'll create the records update form.
success_url: The URL that we'll redirect if the form has been updated successfully.
urls.py
in urls.py, we need to add a path of our UpdateView.
#UpdateView
path('post/<slug:pk>/', Update.as_view(), name='Post_update'),
Posts-list.html
in Posts-list.html template, we need to add these following lines to display the list of our Posts.
<html>
<head>
<title>ListView</title>
</head>
<body>
<h1 style="padding-bottom: 30px">list of posts</h1>
{% for i in object_list %}
<div style="margin-bottom: 40px">
<h4>{{i.title}}</h4>
<a href="{% url 'Post_update' i.id %}">update</a>
</div>
{% endfor %}
<h3><a href="{% url 'create_article' %}">add</a></h3>
</body>
</html>
update_form.html
in update_form.html template, we need to create a form that will update our Posts.
<html>
<head>
<title>UpdateView</title>
</head>
<body>
<form method="POST">{% csrf_token %}
<!-- form variable -->
{{ form.as_p }}
<input type="submit" name="sumbit">
</form>
</body>
</html>
3. UpdateView Result
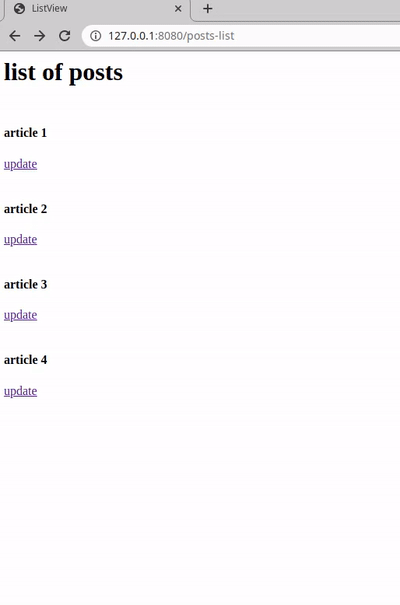
happy Codind