Last modified: Jan 10, 2023 By Alexander Williams
Django Queryset | Sort Queryset by Date
In this post, I will show you how to sort Django Queryset by date by using the order_by() method.
First, we'll create a simple comments model, then sort the model Queryset by date.
Table Of Contents
Create a Comments model
in our models.py, we need to add the following lines.
models.py
class Comments(models.Model):
name = models.CharField(max_length=300)
comment = models.TextField()
date = models.DateField(auto_now_add=False)
Now, let's display our model in the Django admin interface.
admin.py
@admin.register(Comments)
class CommentsAdmin(admin.ModelAdmin):
#list display
list_display = ['name', 'comment', 'date']
makemigraion and migrate our model
Let's add some comments to our model.

2. Sort the model's queryset by date
To sort the model Queryset, Django provides the order_by method.
In the first example, we'll sort our model's Queryset from oldest to newest.
views.py
def comment_view(request):
""" sort comment model by date """
comments_ordering = Comments.objects.all().order_by('date')
return render(request, "comments.html", {"comments":comments_ordering})
urls.py
adding a path for the comments view.
path('comments/', comment_view),
comments.html
in the comments.html file, we need to put these following lines.
<!DOCTYPE html>
<html>
<head>
<title>Sort Django Model By Date</title>
</head>
<body>
{% for comment in comments %}
<div>
<h2>name: {{comment.name}} </h2>
<h2>comment: {{comment.comment}} </h2>
<h2>date: {{comment.date}} </h2>
<br>
<br>
</div>
{% endfor %}
</body>
</html>
result:
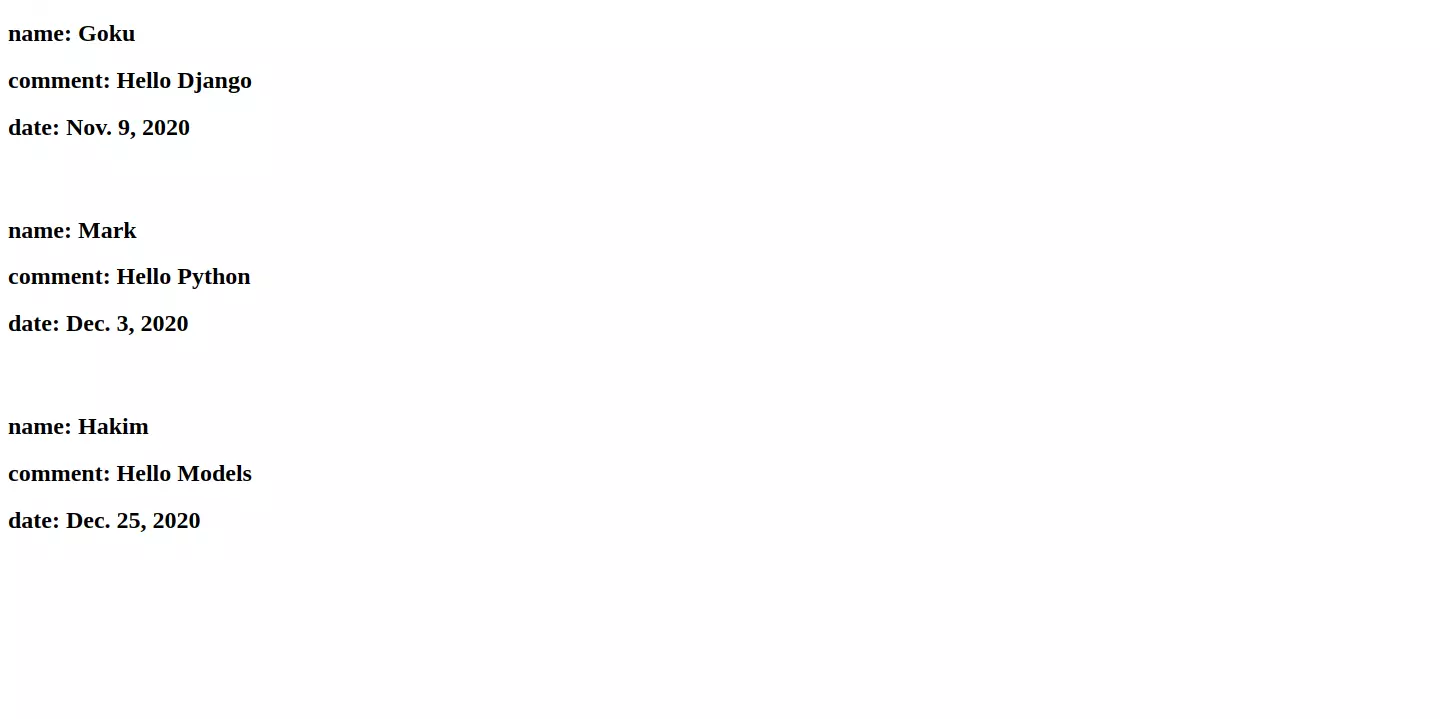
In the second example, we'll sort the model's Queryset from newest to oldest.
views.py
def comment_view(request):
""" sort comment model by date """
comments_ordering = Comments.objects.all().order_by('-date')
return render(request, "comment.html", {"comments":comments_ordering})
result:
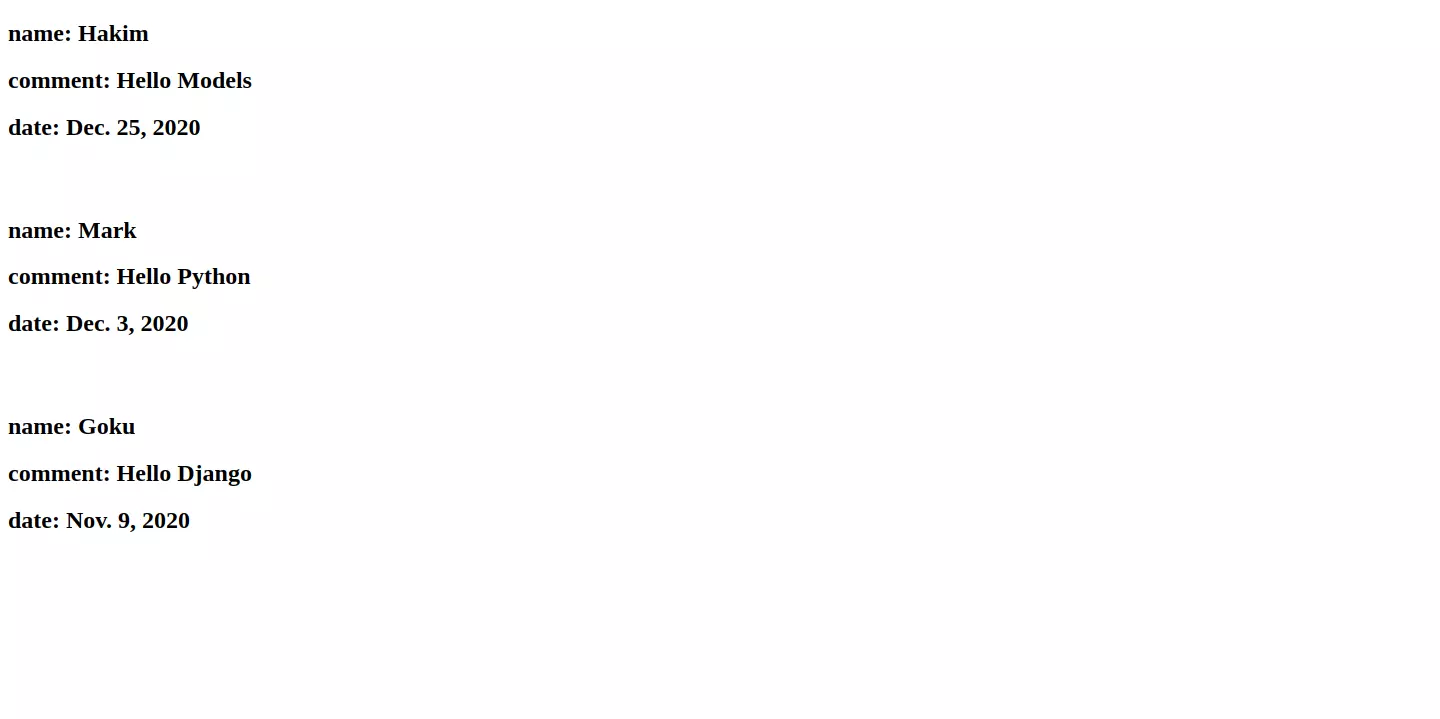