Last modified: Jan 10, 2023 By Alexander Williams
Understand how to use Django CreateView with example
CreateView is a class view that helps you to display a model's form with validation errors and save objects.
To understand more, let's see an example.
Django CreateView Example
First of all, we need to create a model in models.py.
class TestModel(models.Model):
name = models.CharField(max_length=50)
parent = models.CharField(max_length=50)
def __str__(self):
return self.name
Now, let's create CreateView class for our model and display the model's form.
In views.py:
from django.views.generic.edit import CreateView
class TestCreateView(CreateView):
model = TestModel
template_name = 'test.html'
fields = '__all__'
success_url = "/create"
model:the model's name.
template_name: the template that we'll display the form.
fields: the fields that you want to display, ex ['name', 'parent'], __all__ means all fields.
success_url: The URL to redirect to after successful submission of the form.
In urls.py, create a path for our View.
path('create/', TestCreateView.as_view()),
In test.html:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<h1>Test Model Creation</h1>
<form method="post">{% csrf_token %}
{{ form.as_p }}
<input type="submit" value="Save">
</form>
</body>
</html>
Result:
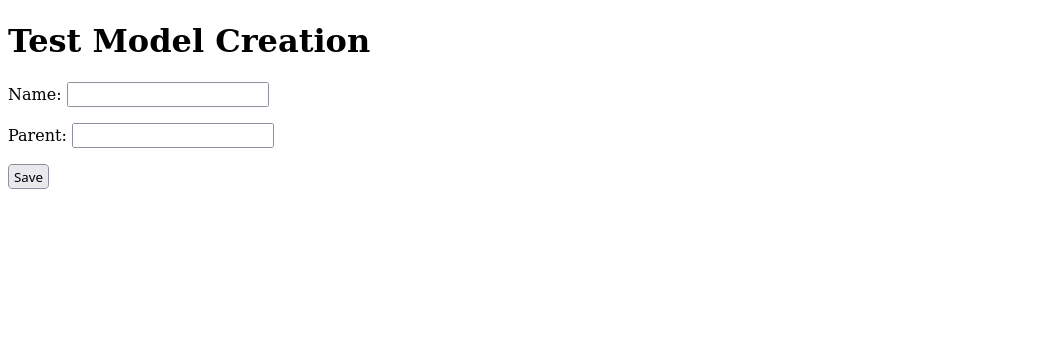
View-source:
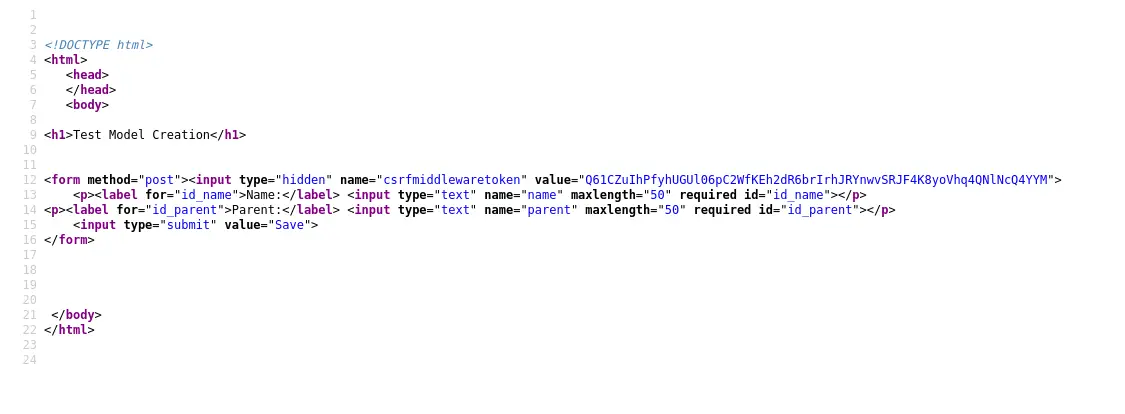
Submission the form:
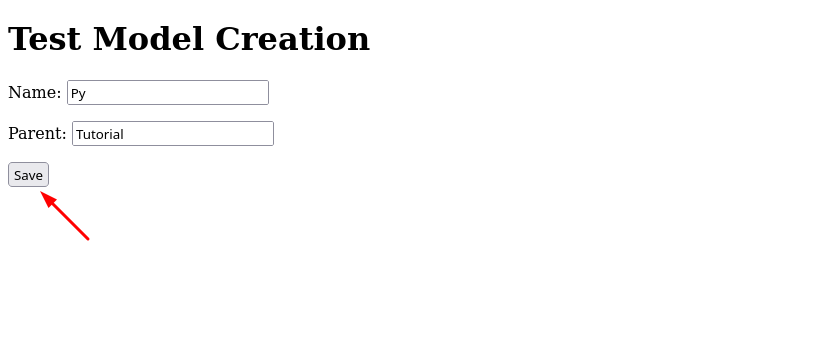
Admin interface:
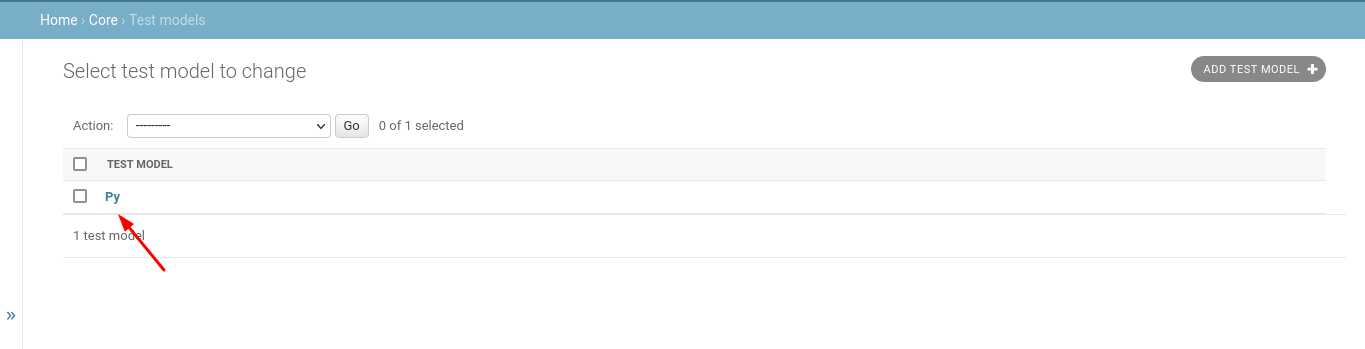